월요일! 🐱🐉
지난 금요일에 이어 PyCharm과 MySQL을 이용해 Django를 배운다!
PyCharm으로 지난번에 했던 Project(MyFirstWeb_0214) 생성, Django 설치 및 MySQL 연동을 똑같이 진행했다.
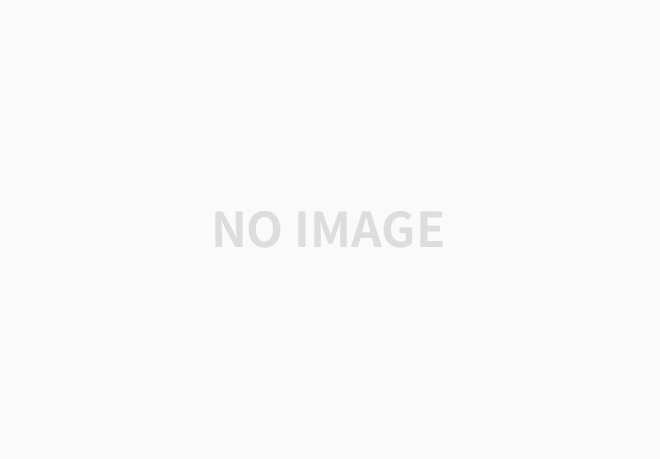
1. MySQL로 DB Table을 만드는 것이 아닌, PyCharm에서 Python 모듈 중 models를 불러와서 class를 만듦
polls 폴더의 models.py 파일 안에 class로 DB Table 명세를 설정함. model class를 만들 때는 반드시 model.Model을 상속해야 함. PK(Primary Key)가 자동으로 하나 설정됨
from django.db import models
class Question(models.Model):
# id = models.IntegerField() # 자동으로 PK column 생성됨
question_text = models.CharField(max_length=200)
pub_date = models.DateTimeField()
class Choice(models.Model):
choice_text = models.CharField(max_length=50)
votes = models.IntegerField(default=0)
# CASCADE는 질문이 지워지면 질문에 대한 항목도 지워라. 관계 제약사항
question = models.ForeignKey(Question, on_delete=models.CASCADE)
2. MySQL DB에 Table을 만들기 위해서는 python manage.py migrate 명령어를 써야 하나,
특정 명세서 기반이 아닌 작성한 class를 기반으로 Table을 만들기 위해서는
python manage.py makemigrations 사용 후 migrate 명령어 써야 함
(MyFirstWeb_0214) C:\python-django\MyFirstWeb_0214>python manage.py makemigrations
Migrations for 'polls':
polls\migrations\0001_initial.py
- Create model Question
- Create model Choice
(MyFirstWeb_0214) C:\python-django\MyFirstWeb_0214>python manage.py migrate
Operations to perform:
Apply all migrations: admin, auth, contenttypes, polls, sessions
Running migrations:
Applying polls.0001_initial... OK
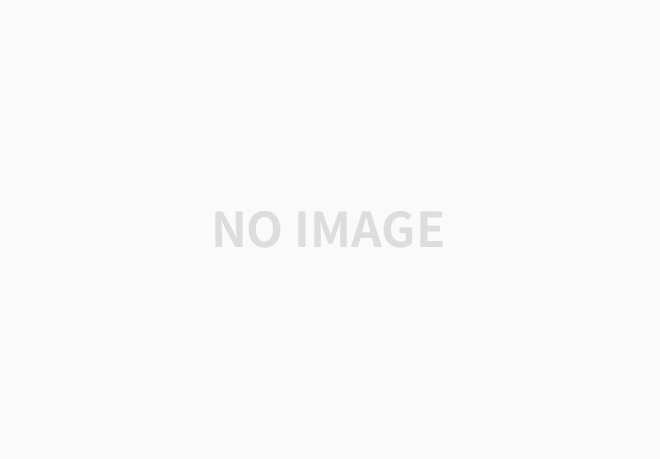
3. 서버 어드민 페이지에서 DB Table을 보려면 PyCharm으로 admin.py에 만들어둔 Model의 class를 등록해야 함
from django.contrib import admin
from polls.models import Question, Choice // 모델 경로
admin.site.register(Question)
admin.site.register(Choice)
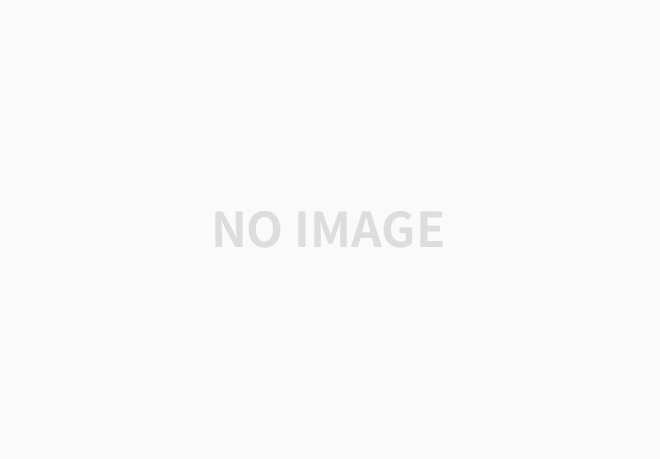
4. Admin Page에서 직접 질문(Column) 추가함
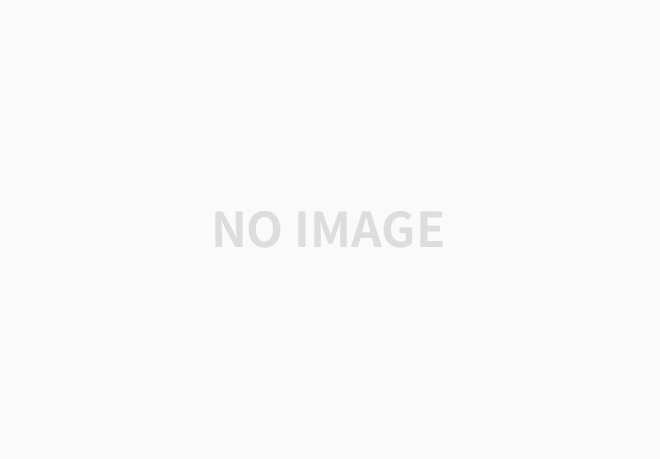
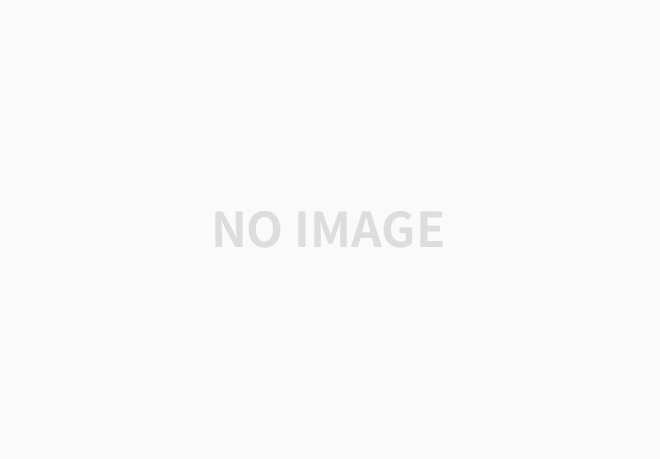
5. Python 객체는 문자열이 아니므로 매직 펑션(def __str__(self): return self.~_text)을 사용해야 어드민 페이지에서 질문을 확인할 수 있음
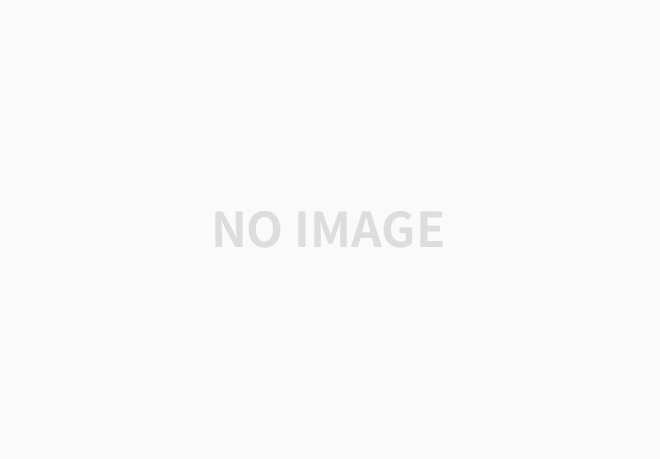
6. Admin Page에서 직접 선택 추가함(+ PK 설정)
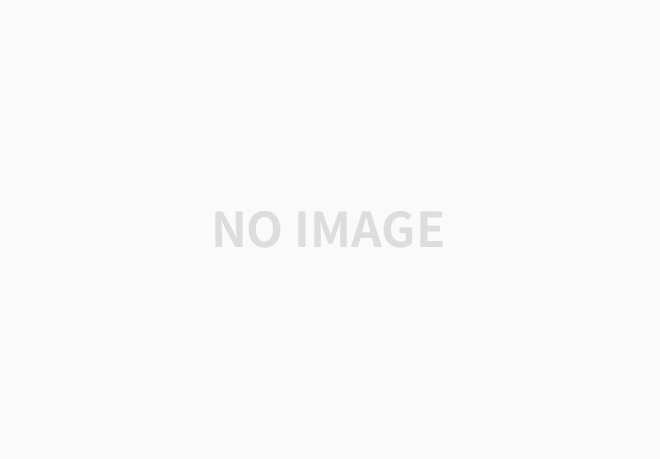
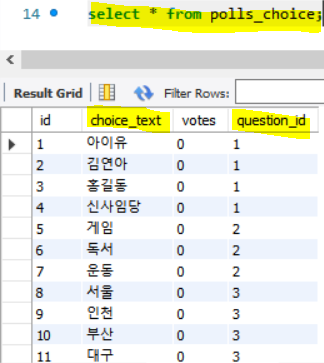
7. Root URLConf 안에서 모든 URL을 다 처리할 수 없기 때문에, 각 application에 대한 URL을 배정해줘야 함↓
mysite 폴더 안의 urls.py(Root URLConf)에 polls App에 대한 URLConf의 경로를 설정함
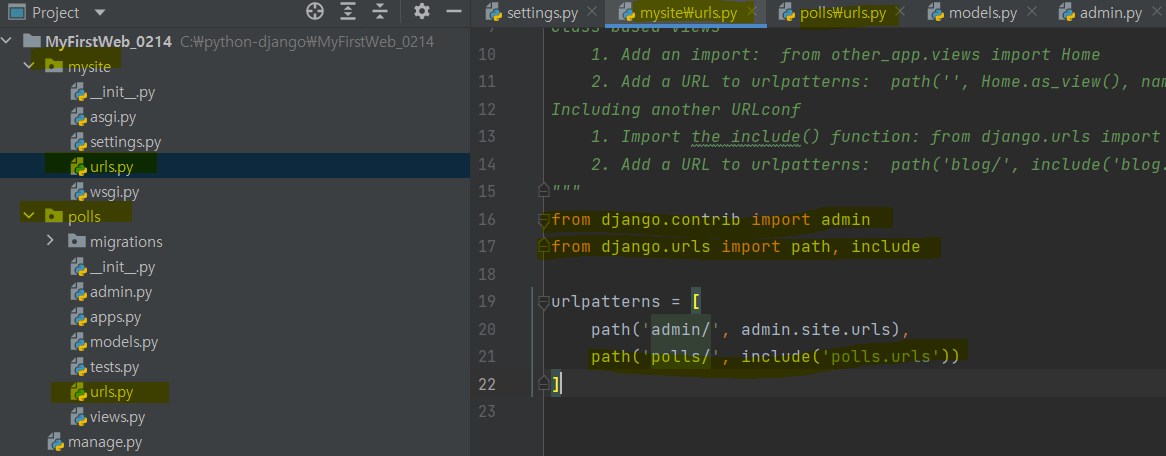
8. urls.py(Root URLConf) 파일을 복사해서 polls App 폴더에 붙여 넣고, 해당 URLConf의 경로를 설정해야 함
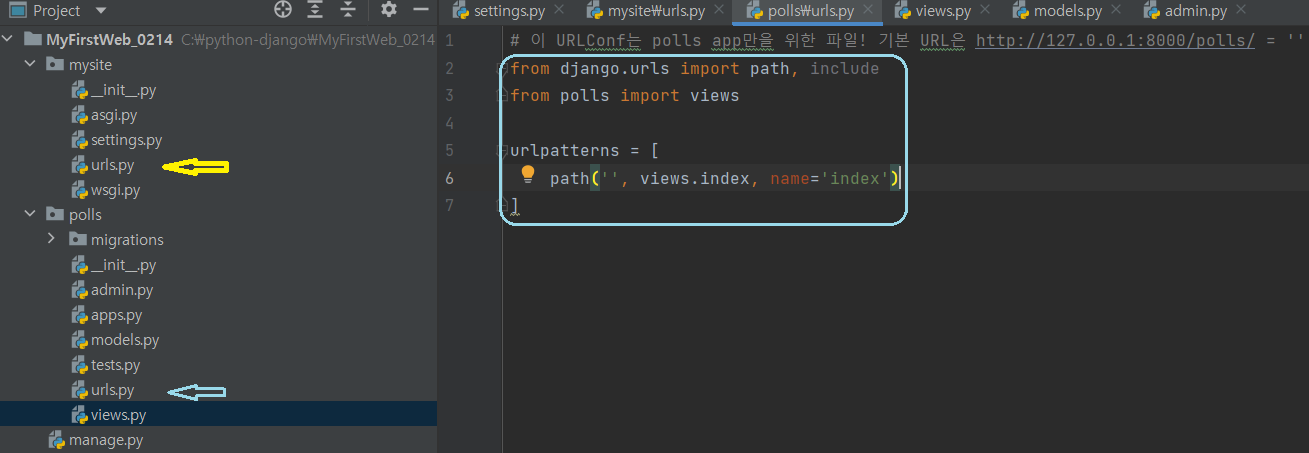
9. polls App에 해당되는 URL로 진입(request)하면 view로 index함수가 호출되므로 이를 정의해야 함
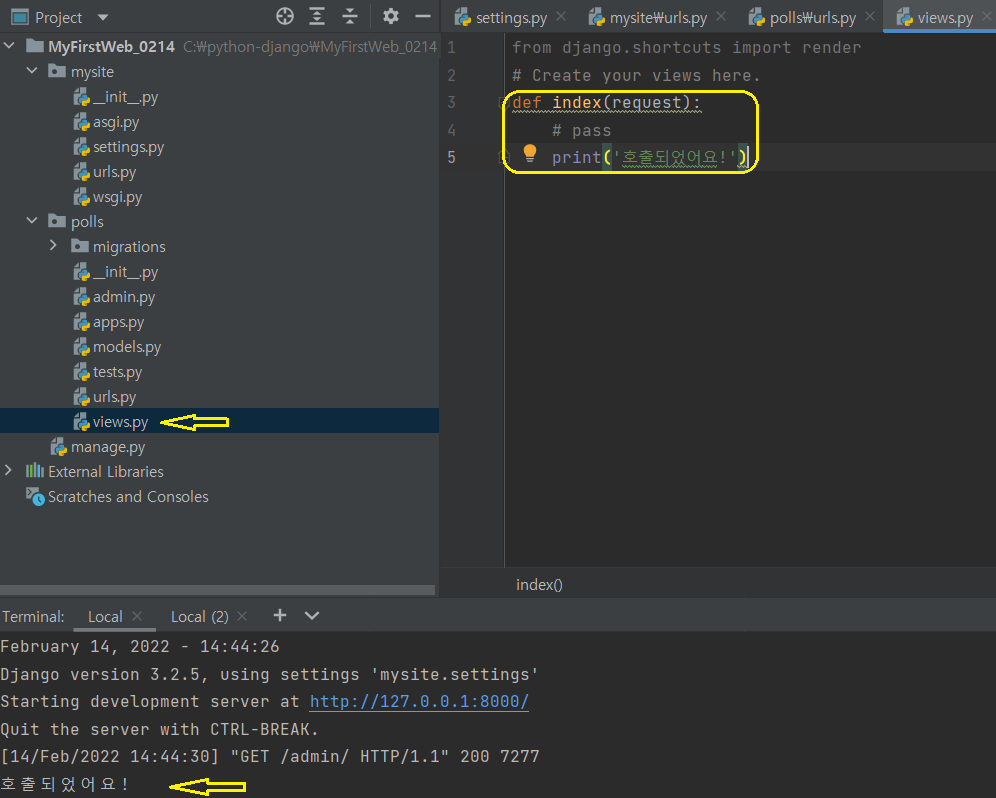
아직 URLConf, View만 설정했기에 Server polls page로 들어가면, Template(response가 none)이 없어 오류창이 뜸
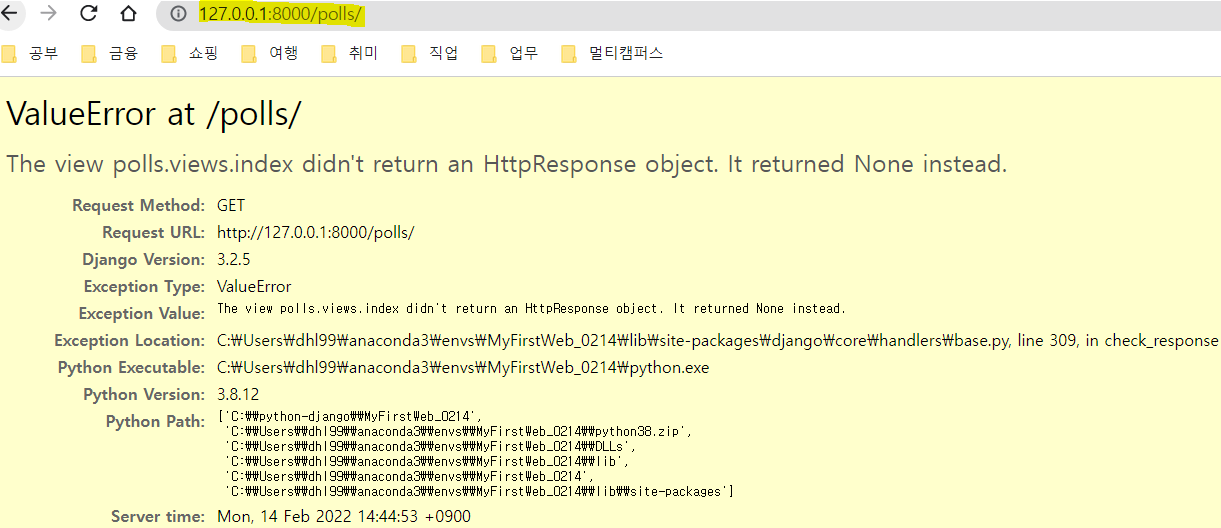
10. Template를 이용해서 client에게 response를 돌려줌
render() 함수는 Template HTML을 이용해서 HttpResponse 객체를 생성함
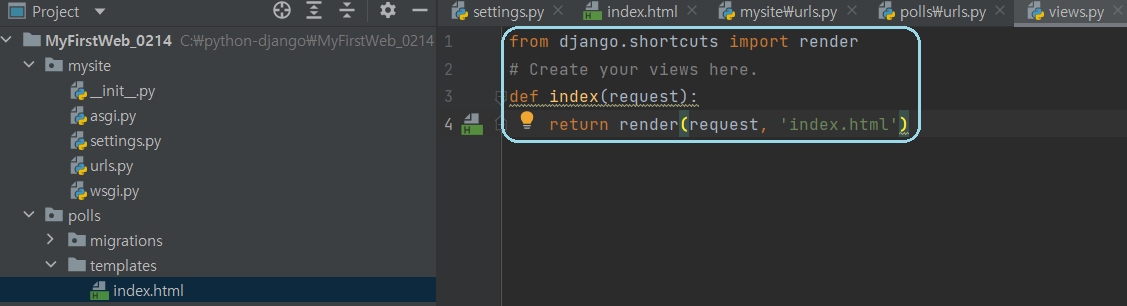
11. polls 폴더 안에 templates라는 신규 폴더를 생성하고, 그 안에 index라는 HTML 파일을 만들어줌
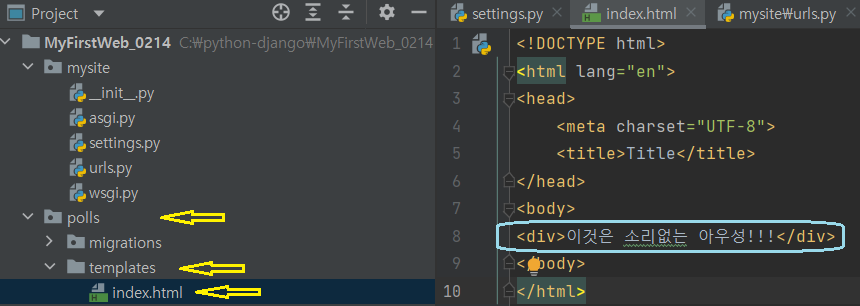
12. Template HTML을 이용해서 HttpResponse 객체만 돌려주는 것이 아니라, dictionary 만들어 추가할 수 있음
Template Tag : HTML 파일에 {{key}} 기입해서 value 가져오기 → 값만 표현
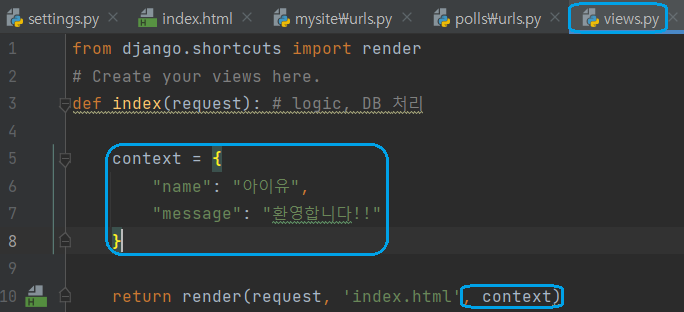
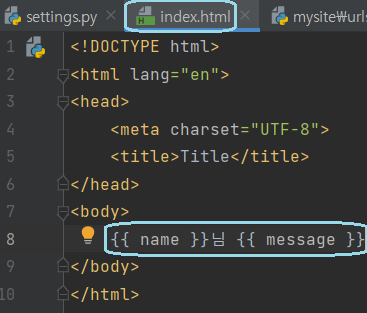
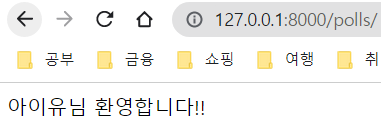
13. Template Tag : HTML 파일에 {% python 반복문 %} 기입해서 DB record 가져오기 → DB 표현
반드시 {% %} 뒤에 {% end %}로 구문 닫아줘야 함
↓polls_views.py↓
from django.shortcuts import render
from polls.models import Question
def index(request): # logic, DB 처리
# Question의 모든 객체를 들고와서, 발행일시 최근순으로 정렬하라
question_list = Question.objects.all().order_by('-pub_date')
context = {
"q_list": question_list
}
return render(request, 'index.html', context)
↓templates_index.html↓
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<ul>
{% for question in q_list %}
<li>{{ question.question_text }}</li>
{% endfor %}
</ul>
</body>
</html>
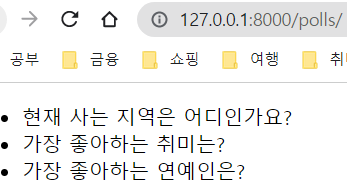
14. namespace : app 마다 URLConfig가 다르므로, Template 또한 구분해주기 위해 urls.py 안에서 구분자 역할을 함. app_name이라는 변수를 이용
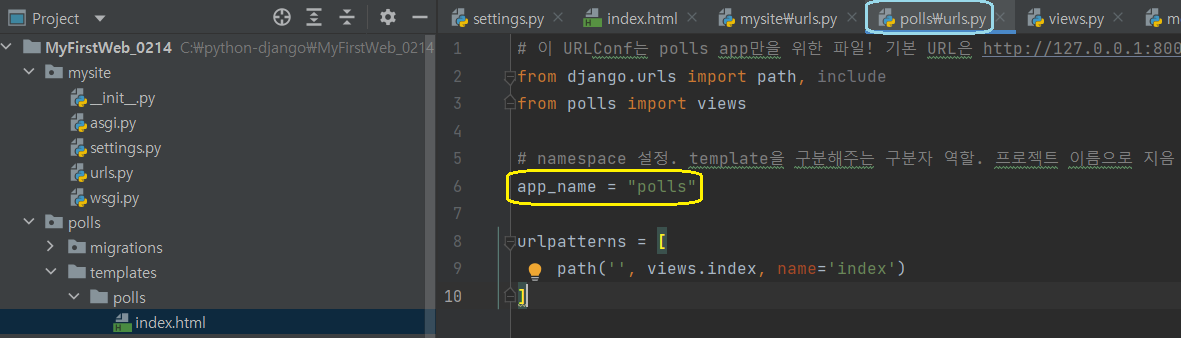
https://docs.djangoproject.com/ko/4.0/intro/tutorial01/
첫 번째 장고 앱 작성하기, part 1 | Django 문서 | Django
Django The web framework for perfectionists with deadlines. Overview Download Documentation News Community Code Issues About ♥ Donate
docs.djangoproject.com
1. 1/17 월 | 2. 1/18 화 | 3. 1/19 수 | 4. 1/20 목 | 5. 1/21 금 |
취업 특강 | 파이썬 환경 설정 (파이참, 아나콘다, 주피터 노트북) |
파이썬 데이터 타입 (list, tuple) |
파이썬 데이터 타입 (range, string, dictionary, set, bool) |
파이썬 사용자 정의 함수, 객체지향, 사용자 정의 클래스 |
6. 1/24 월 | 7. 1/25 화 | 8. 1/26 수 | 9. 1/27 목 | 10. 1/28 금 |
파이썬 사용자 정의 클래스, 사용자 정의 모듈 |
DB(MySQL) DBMS, MySQL 환경 설정, DB/테이블 생성, DB 입력·활용, Index |
DB(MySQL) View, 백업과 복원, DB 모델링, 관계(PK, FK), DML(CRUD), 데이터 타입 |
DB(MySQL) DML(CRUD), WHERE 조건, 패턴 매칭, SubQuery, 정렬, 그룹핑, 집계 함수, 트랜잭션(ACID 특성) |
DB(MySQL) JOIN, UNION, NOT IN, WEB |
11. 2/3 목 | 12. 2/4 금 | 13. 2/7 월 | 14. 2/8 화 | 15. 2/9 수 |
WEB WebStorm 환경 설정, HTML, CSS, JavaScript, jQuery CDN |
WEB jQuery 문법, Selector, Method |
WEB jQuery Method, Event, AJAX |
GitHub 특강 소스코드 관리, Fork, Clone, Branch, Commit, Push, Pull-Request, Rebase |
GitHub 특강 프로젝트 관리, Fetch, Rebase, Merge, Conflict |
16. 2/10 목 | 17. 2/11 금 | 18. 2/14 월 | 19. 2/15 화 | 20. 2/16 수 |
WEB jQuery AJAX, Bootstrap, Open API |
Django WSGI, MVC, MVT, ORM |
Django MVT, ORM |
* 전체적인 구조를 이해하자!